Part 2 – Error Handling Best Practices in Angular
- Posted by Muthukumar Dharmar
- Categories Angular, Best Practices
- Date July 7, 2020
In Part 1, I have explained the typical error handling approach and best practices for error handling in the Angular web. In this article, we will implement what we discussed in the previous post. So, let’s start with our sample project.
Demo
I have stimulated both Client & Http Response error in this demo. Let’s see the exception first, then we will go through the step by step implementation process on how to handle this exception globally.
Client-Side Error
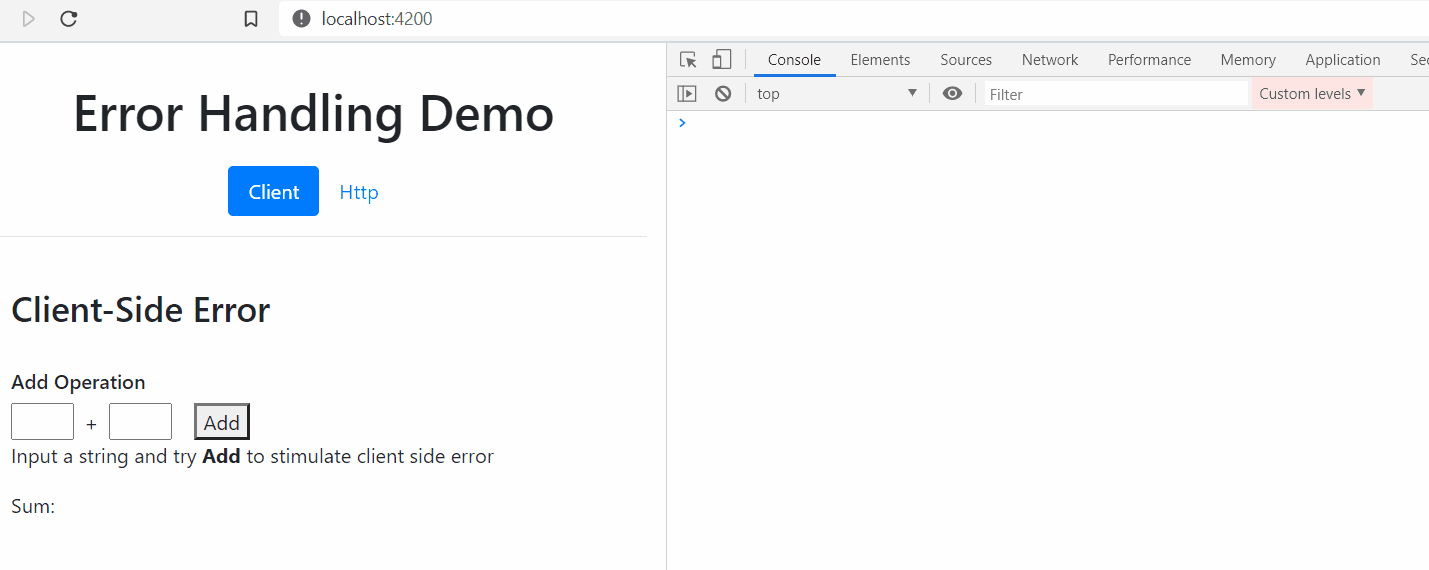
Http Error
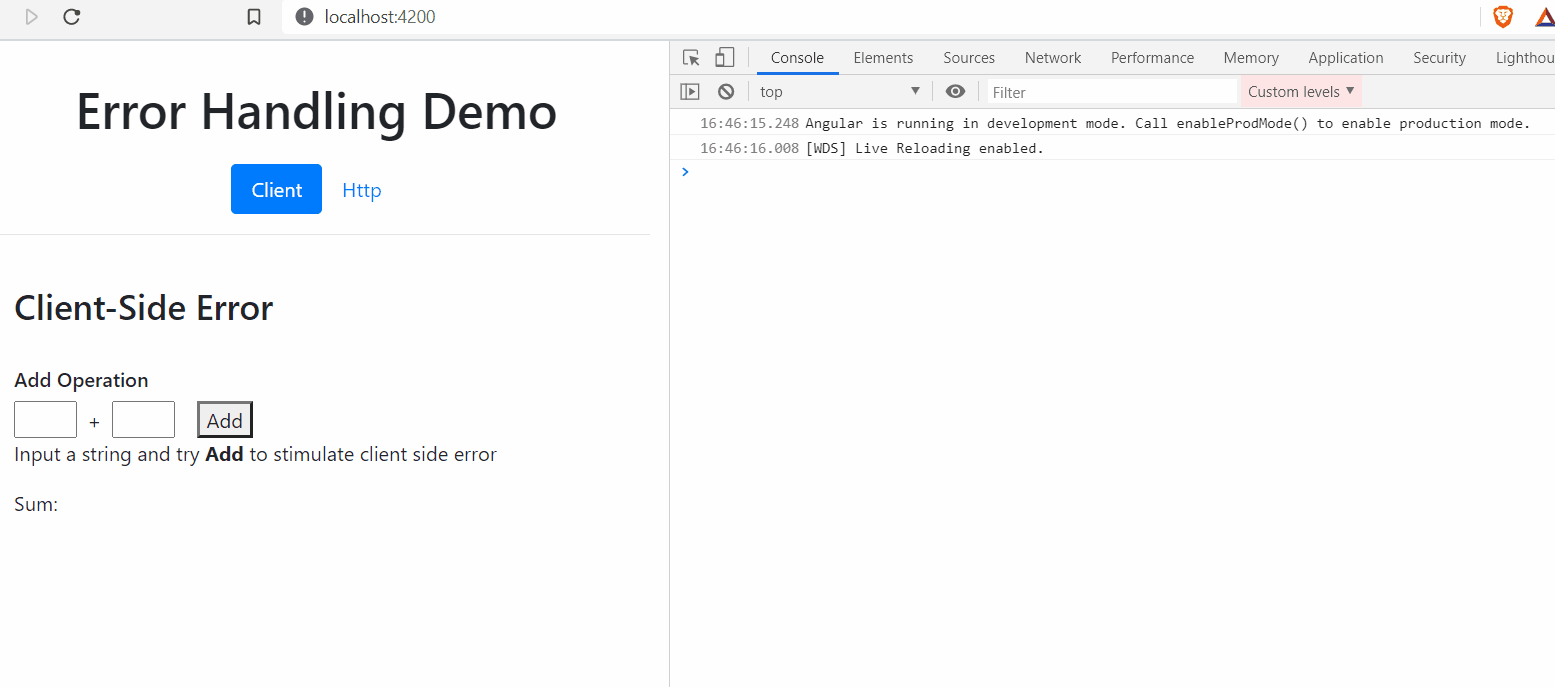
Now we have seen the exception in action, lets start the implementation to handle these exceptions globally.
Project Structure
This is a typical angular project created using Angular-CLI, lets briefly discuss the key components.
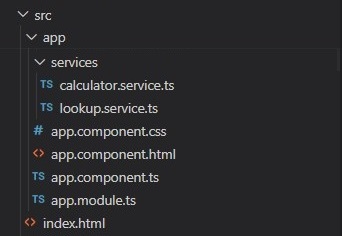
App component is our UI, where we have a tab implementation to navigate between two simple views (refer above demo).
CalculatorServervice is a simple service that holds the add logic.
LookupService is the service that is communicating to a public Restful API and returns the country info based on the search text.
Its time to look into source code
See the below html content from app.component.html, where we have a tab implementation to navigate between two simple views.
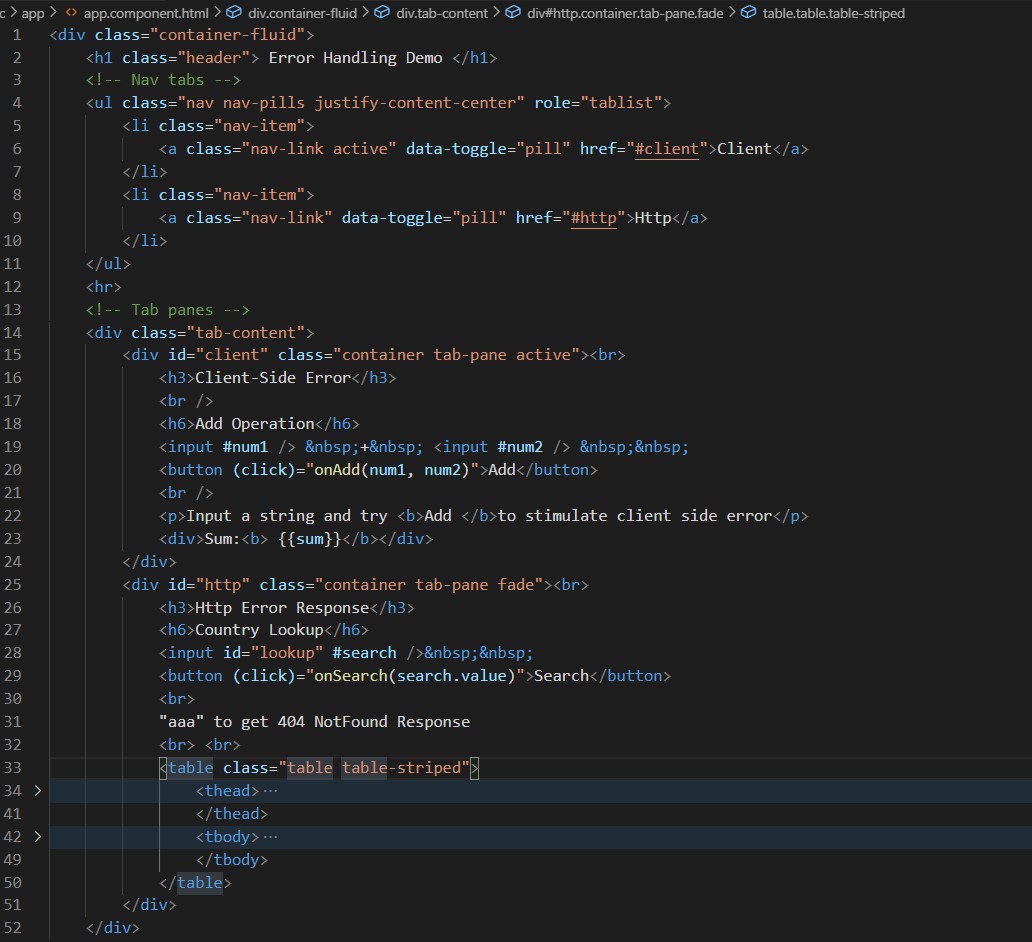
Below is the code behind app.component.ts file that has the methods to execute during user interaction.
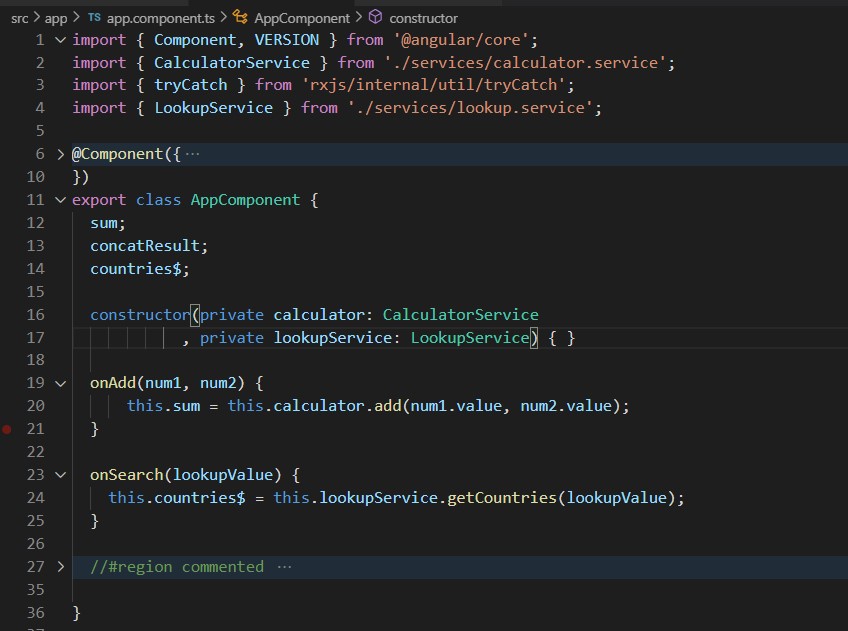
Finally, below are the services which holds the actual implementation.
Calculator service which has the Add function with a type check on input parameters and throws an Invalid Input exception.
Lookup service uses a free restful API solution to fetch the countries list. Thanks to the REST COUNTRIES project and the free restful services provided for the developers.
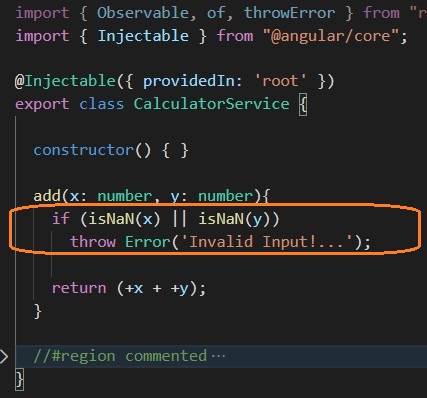
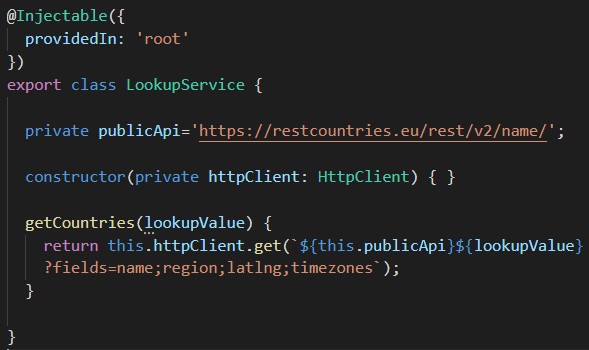
Handling Errors in Angular Way
Let implement the GlobalErrorHandler class and have a code walkthrough 🙂
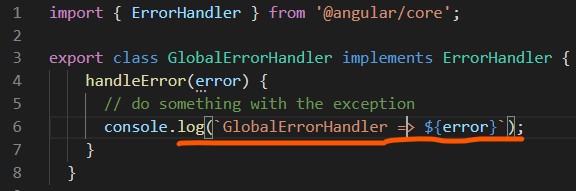
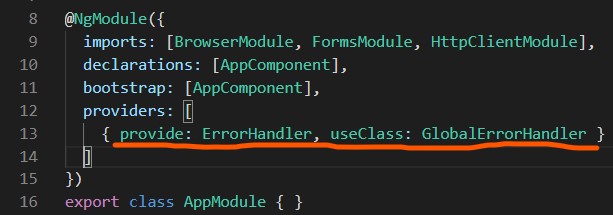
In GlobalErrorHandler class, we have inherited ErrorHandler from @angular/core and implemented handleError method, which captures all the exceptions and then logs the error into the developer console.
In AppModule, we have included the provider statement in @ngModule segment, so that our application can use our GlobalErrorHandler as the default ErrorHandler.
Lets run the app and see how it works
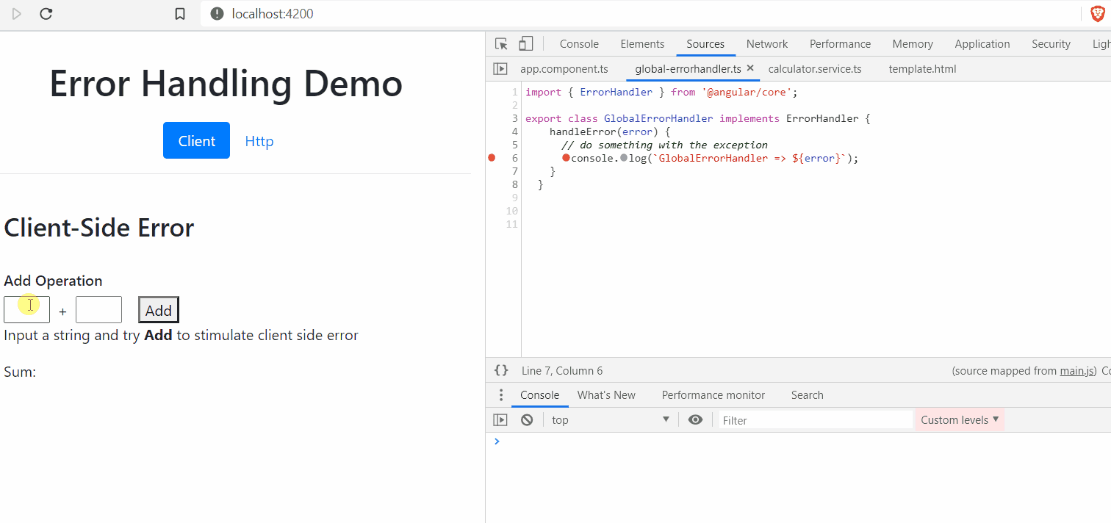
Now you have seen our global error handler is capable of capturing all the exceptions that occur in our application including the HttpErrorResponses. Let’s make a few changes to handle client and Http Error separately.
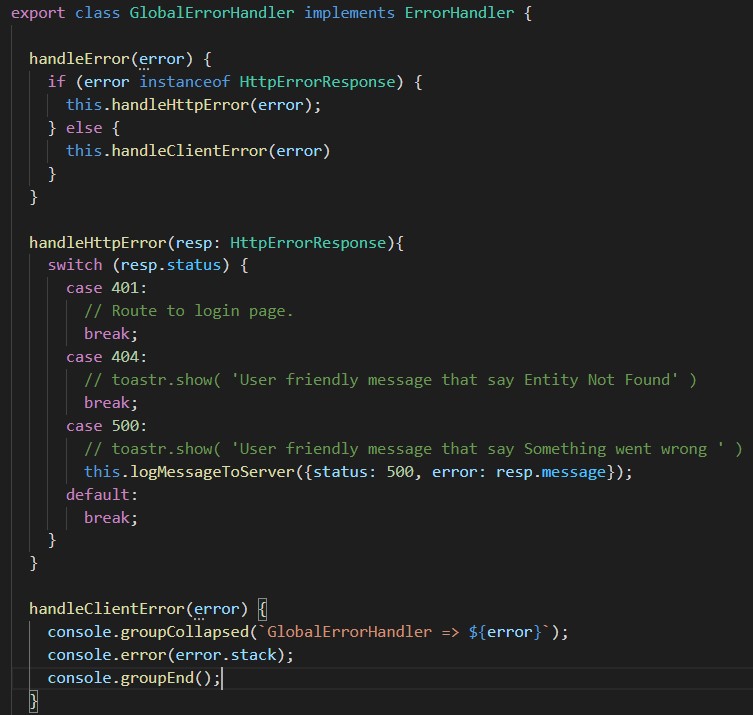
We have introduced a condition in our handleError method, so that it will invoke different methods to handle client or server-side errors.
The handleClientError method is simply logging the errors in the developer console, but in real-time we may have to send the error info the loggerApi to persist in the database
The handleHttpError method has a switch statement to isolate and react based on the response status.
Lets see final demo
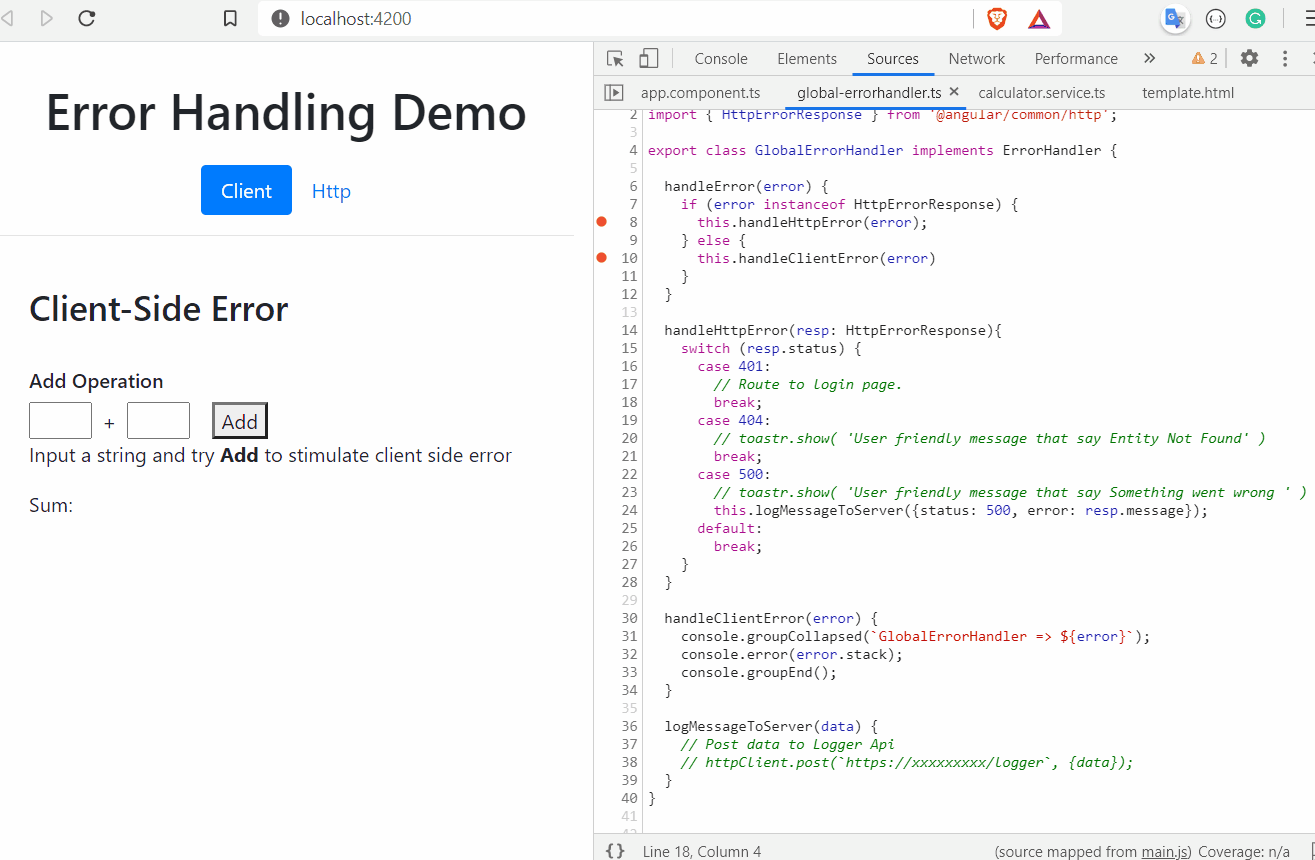
Here we have separated Client & Http Errors, not only the separation we are also handling the Http Errors based on the status code.
Yes, for Http errors I have written some pseudo-code, I guess this is sufficient since my objective is to provide you a standard solution to handle errors in your angular applications. In real-time we can handle this based on the business need.
PROPOSED SOLUTION:
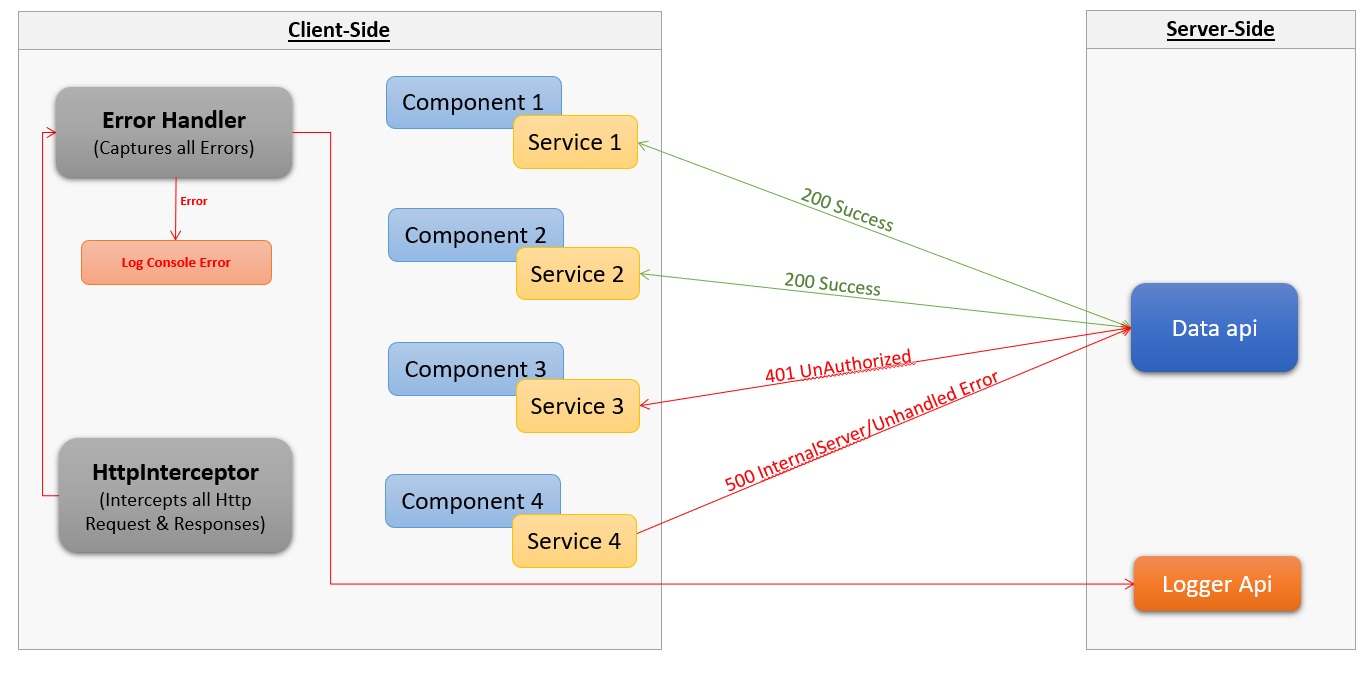