Part 3 – Error Handling Best Practices in Angular
- Posted by Muthukumar Dharmar
- Categories Angular, Best Practices
- Date July 26, 2020
In Part 2, We have implemented our Global Error Handler class and handled all the client-side & Http errors in our application globally. At the end of Part 2, we had a question why do we even have the HttpInterceptor object in our proposed solution, RIGHT !…
Let’s jump into the article to understand the advantage of HttpInterceptor.
Typical Http Request / Response Cycle in Angular
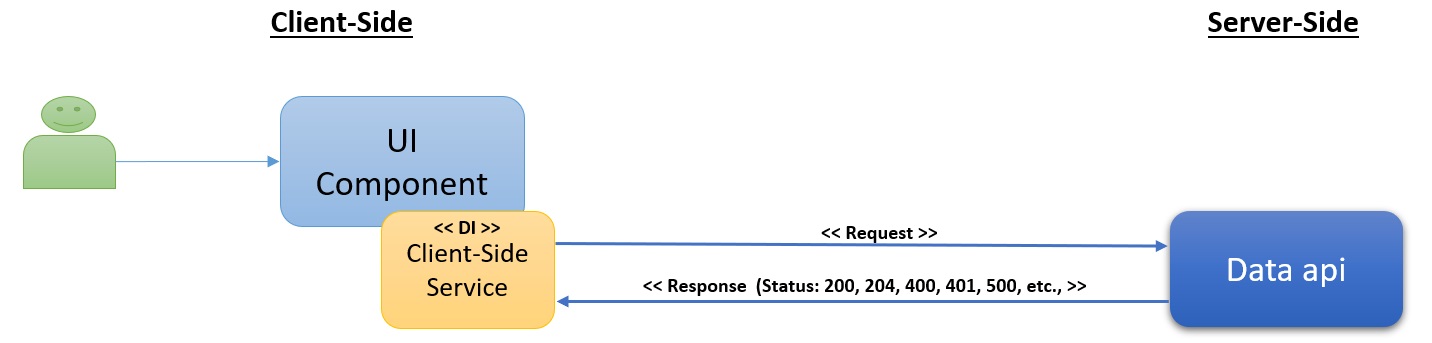
On User interaction, an Http request is being invoked by the client application and the API from Server-side processes the request and returns the Http response.
This response could be of status:
- 1xx (Informational): The request was received, continuing process
- 2xx (Successful): The request was successfully received, understood, and accepted
- 3xx (Redirection): Further action needs to be taken in order to complete the request
- 4xx (Client Error): The request contains bad syntax or cannot be fulfilled
- 5xx (Server Error): The server failed to fulfill an apparently valid request
Http Errors
Http errors can be of two types (ref: angular docs)
1. Error response returned from backend, Lets say the server has successfully received the request but for some reason, it couldn’t process/return a successful response.
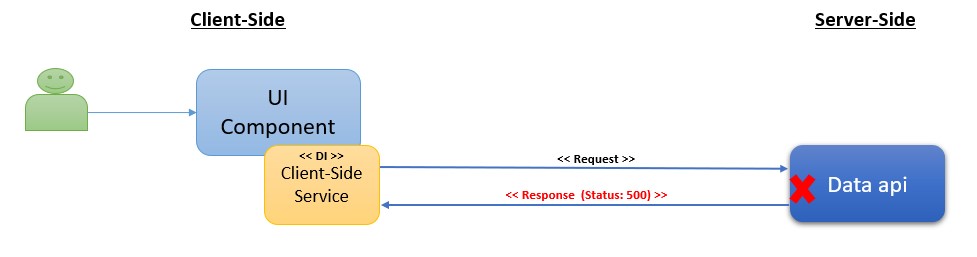
This could be:
- 401 – UnAuthorized, 404 – Resource Not Found, 400 – Bad Request
- 500 – Internal Server Error, etc., (ref.: Status Codes)
2. Something from the client-side causing the request to fail. The exception that happens in the client-side during the Http request/response process. Hence this will be handled by HttpClientModule and the error will be wrapped as a HttpErrorResponse that includes error property of type ProgressEvent.
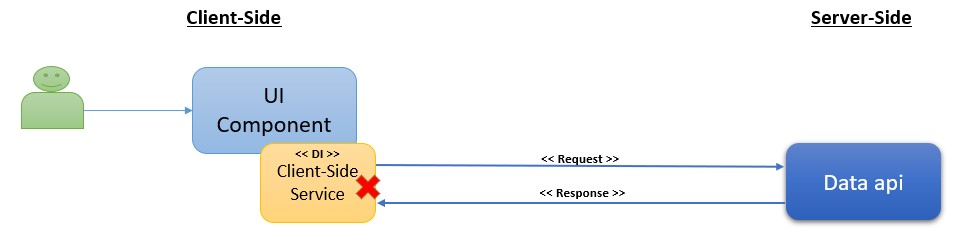
- A network error
- An exception happened in the request process stream.
- An exception is thrown in RxJs operators.
Error handling scenarios
- For server-side errors, its a best practice to handle those errors from the API. In other words, those exceptions should be logged by the WebAPI itself. Hence the client doesn’t need to make a call to log server-side errors.
- Only the client-side errors/activity trace can be logged by the client application using the logger API.
Advantages of HttpInterceptor
Below are a few cases where we can take advantage of HttpInterceptor.
Add/Update Request Header – we can intercept and add Authorization header with auth token to every outgoing request.
Cache the request and response – we can write a caching interceptor to implement caching mechanism and serve certain requests from the local cache to improve performance.
Progress indicator – we can even show/hide the progress indicator by intercepting the request and response.
Retry Failed Request – some errors like network interruptions will automatically go away and return a successful response if we try again. It would be better, If we could automatically retry the failed request before passing it to ErrorHandler, right!… Actually we can do this with the help of HttpInterceptor & the RxJs retry operator.
Handling Errors in Angular Way
Let implement the GlobalHttpInterceptor class and have a code walkthrough 🙂
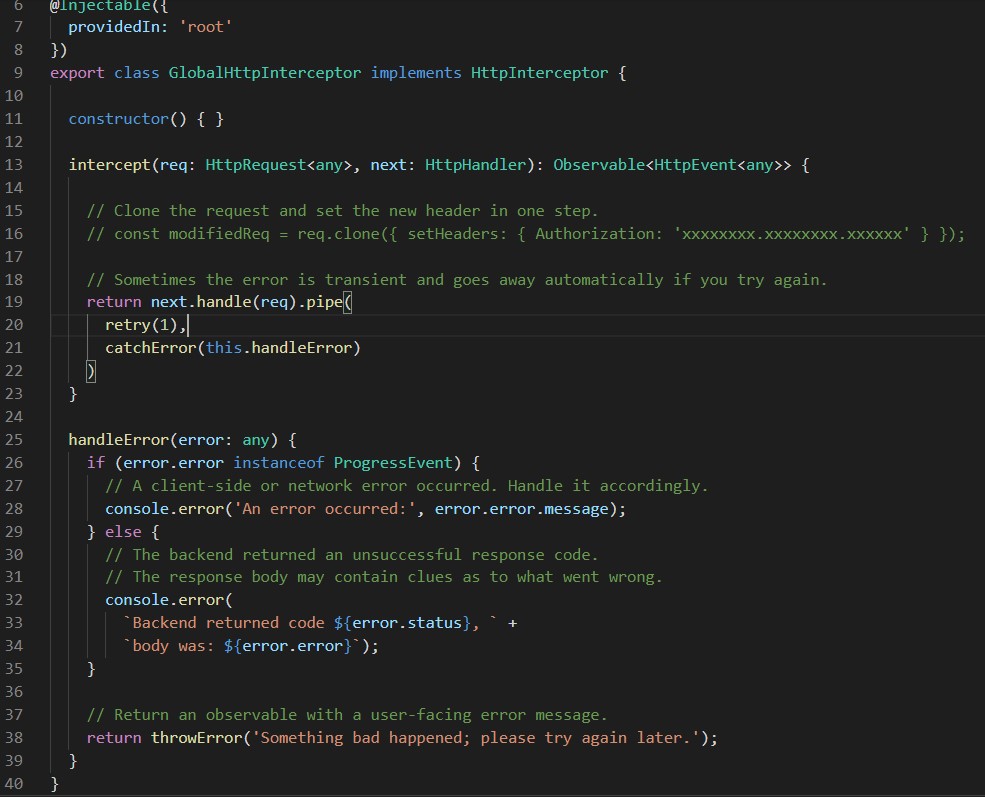
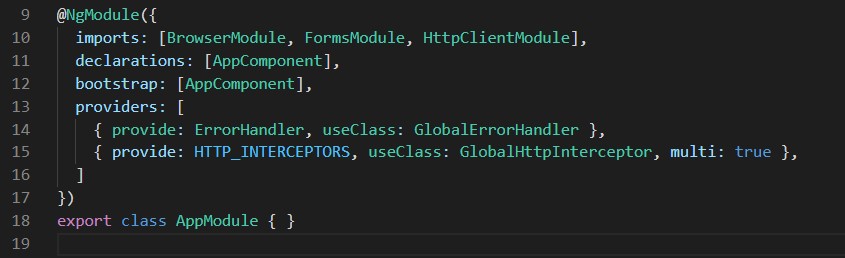
Here our objective is to isolate all the exceptions that occurred during the HTTP request/response pipeline. Now we can move our error handling logic for HTTP status codes from the GlobalErrorHandler to this Interceptor and handle all the HTTP related errors from here.
I have also included the code sample(line# 16) to add an Authorization header to all the outgoing requests.
And If you notice, I have added the retry() operator in the request pipeline, so that when there is an error response then it will try executing the request once again.
This is just to give you an idea about the advantage of HttpInterceptor, but in real-time you should not retry all the requests. Instead, we can implement retryWhen() operator to decide and retry based on the business need. One example scenario would be, status code of 500 Internal server can implement retry with some delay.
PROPOSED SOLUTION:
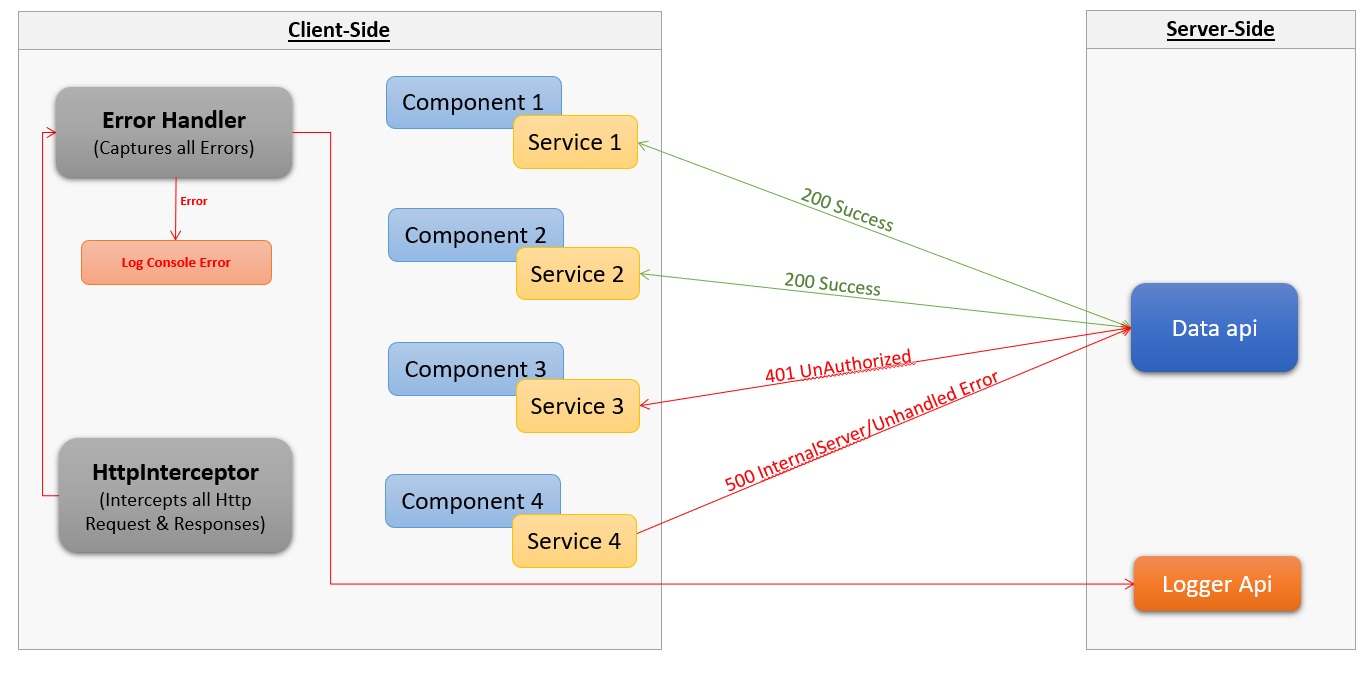
I hope this Error Handling Best Practices in Angular series was useful for everyone 🙂
Next post