Angular – Display image from an API response
- Posted by Muthukumar Dharmar
- Categories Angular
- Date July 31, 2021
In this short article, we will have two different approaches to display an image that’s been fetched from an API response.
The requirement is to display a default placeholder image, then at runtime based on the availability, we will replace the image that was received from an API response.
Angular Pipe Approach
Below pipe will fetch the image data based on the data bound to the element.
@Pipe({ name: 'thumbnail', }) export class ThumbnailPipe implements PipeTransform { constructor(private imageSvc: ImageService, private sanitizer: DomSanitizer) { } transform(value: any, args?: any): any { return this.imageSvc.getBase64Image(value) .pipe(map((baseImage: any) => { let objectURL = 'data:image/jpeg;base64,' + baseImage.image; return this.sanitizer.bypassSecurityTrustUrl(objectURL); })); } }
Usage in html
<h3> Display image using Pipe </h3> <img id="myimage" [src]='(image | thumbnail) | async' />
Angular Directive Approach
In this example, we have a default placeholder image already bound to our <img> element, which will be replaced with the data received from API, if any.
@Directive({ selector: '[thumbnailImage]', }) export class ThumbnailImageDirective { @Input() key = null; constructor(private elementRef: ElementRef, private imageSvc: ImageService) { } ngOnInit() { this.imageSvc.getBase64Image(this.key) .subscribe((baseImage: any) => { let objectURL = 'data:image/jpeg;base64,' + baseImage.image; setTimeout(() => { this.elementRef.nativeElement.src = objectURL; }, 100); }); }
Usage in html
<h3> Display image using Directive</h3> <img id="myimage" [key]="1" thumbnailImage [src]='image' />
Demo
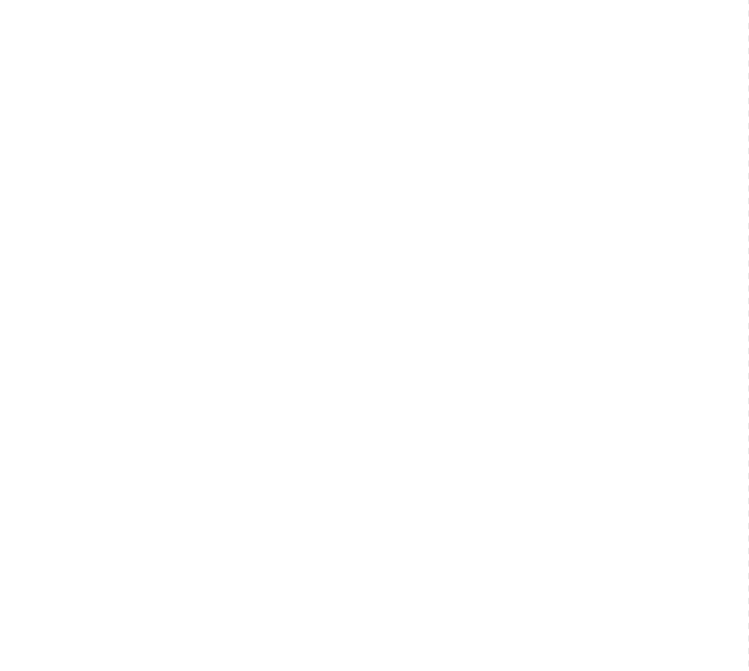
Over 17 yrs of experience in the IT industry.
Skill Set: Angular, RxJS Reactive Programming, AspNet WebApi, WCF, WPF, Azure, etc.,