Angular CLI – Unit Test setup & Code coverage
- Posted by Muthukumar Dharmar
- Categories Angular, Tips & Tricks
- Date June 19, 2020
This article is for angular developers who are already familiar with Angular CLI command-line interface tool, others please refer this and continue
Angular Cli - Unit Test Setup
Angular CLI takes care of all the heavy liftings and it automatically configures all the necessary packages for us.
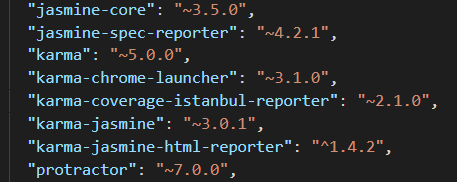
CLI uses:
- Jasmine as the test framework
- Karma as a test runner
And also It has included the packages for coverage & reports.
Important configuration files for Unit Tests.
- karma.conf.js Partial configuration file for karma runner, CLI builds the complete config at runtime.
- test.ts angular CLI uses this test file as a bootstrap for unit test
Since Angular CLI already configured all the necessary setup, we can simply run the below command to execute the tests
- ng test

Let’s say, this is our project structure
- Components contain all the root level angular UI components
- The core module is where we have all core services and utility classes
- feature-modules folder contains all other modules for each features
- shared module that holds the common shareable components, services, etc.,
Now let’s see how karma knows about the test(spec) files that need to be executed.
test.ts where we configure this, In the image below we are creating the context object with all the spec.ts, at runtime these files will be executed by Karma runner.
In this case, all the .spec.ts files in our root folder will be executed by the Karma runner.
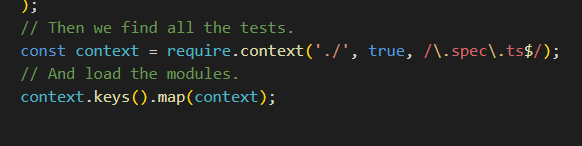
Now we understood the configurations. lets run command ng test to see the output
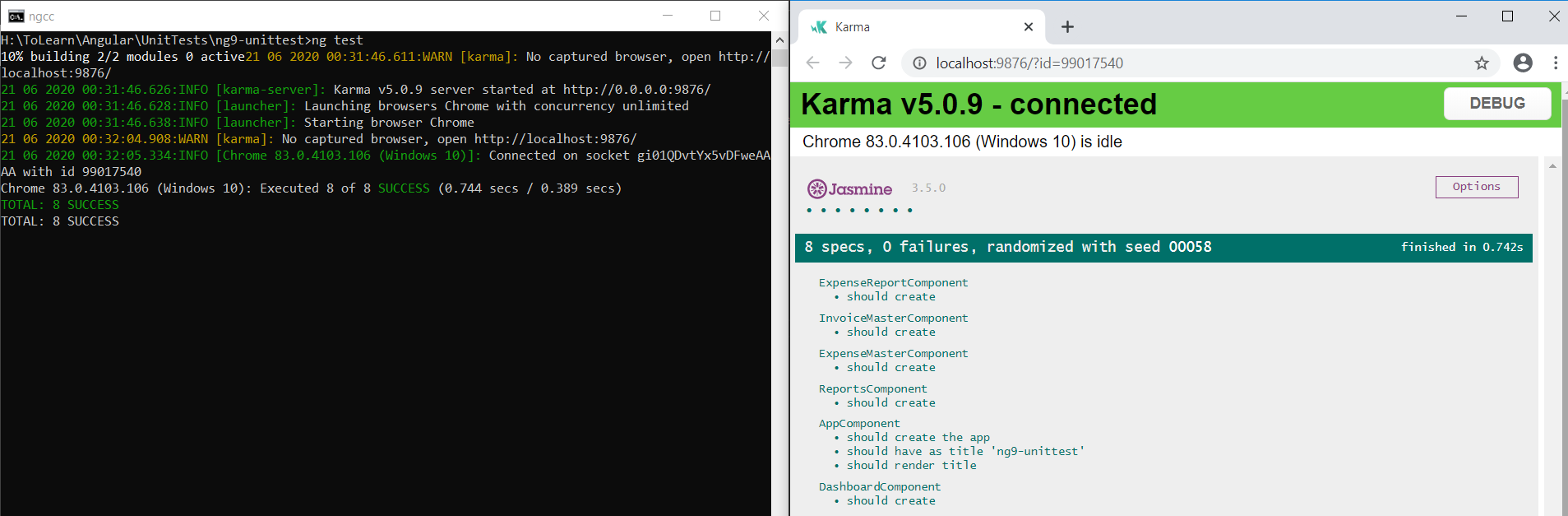
We have successfully run our unit tests using the command ng test. Karma runner has launched a browser instance and ran our tests and it shows a pretty neat view of the test cases execution summary with # of cases executed & # of cases that been failed.
This might be enough for some small applications, but in large enterprise projects this is not sufficient, we need to know how much of test cases we actually covered and what is the success percent, etc.,
Let's configure and generate simple text summary.
Update karma.conf file by including ‘text-summary‘ in reports.
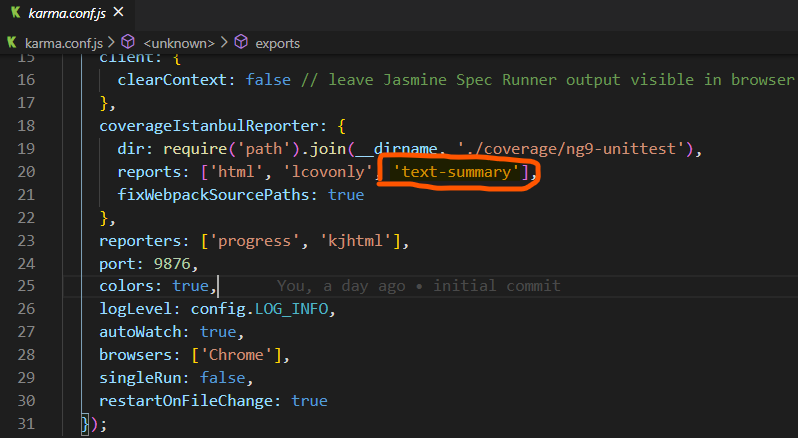
Run our ng test command with –code-coverage option
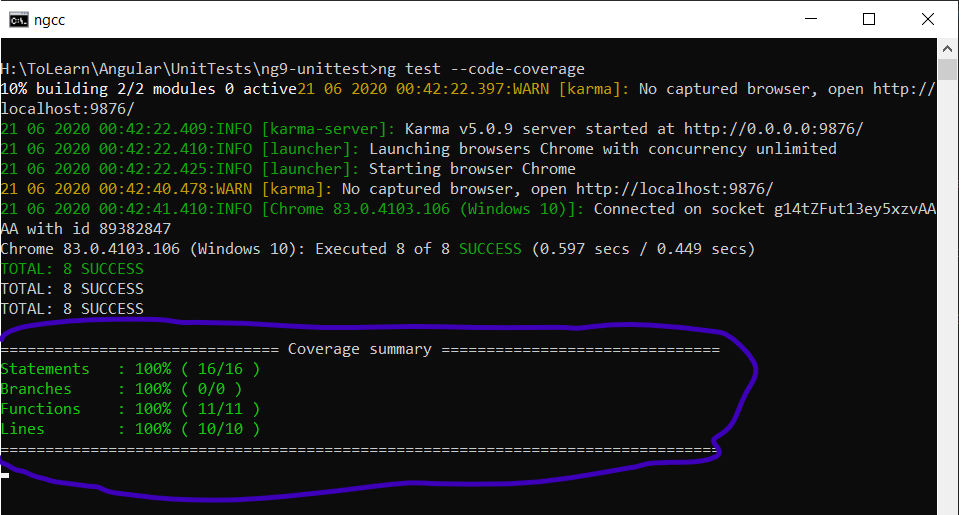
This time we get nice Coverage Summary at the end console view, Cool 🙂
Code coverage report in html
Now let’s make this even better, by generating an HTML report, which can explain the unit test coverage in detail.
Update karma.conf by including global thresholds & reporters
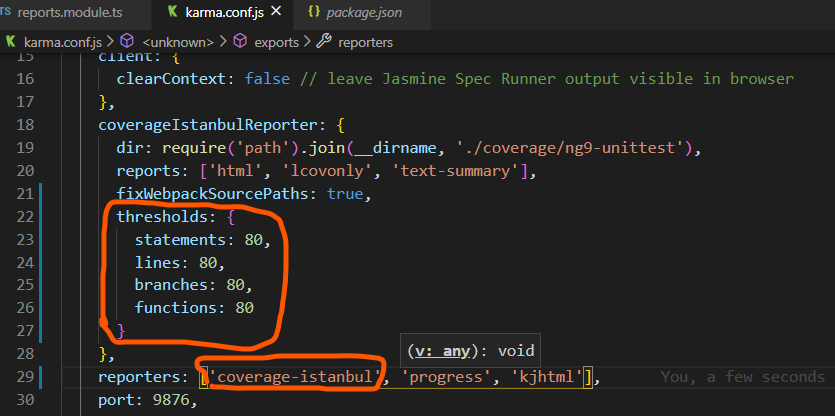
Run the command ng test –code-coverage again
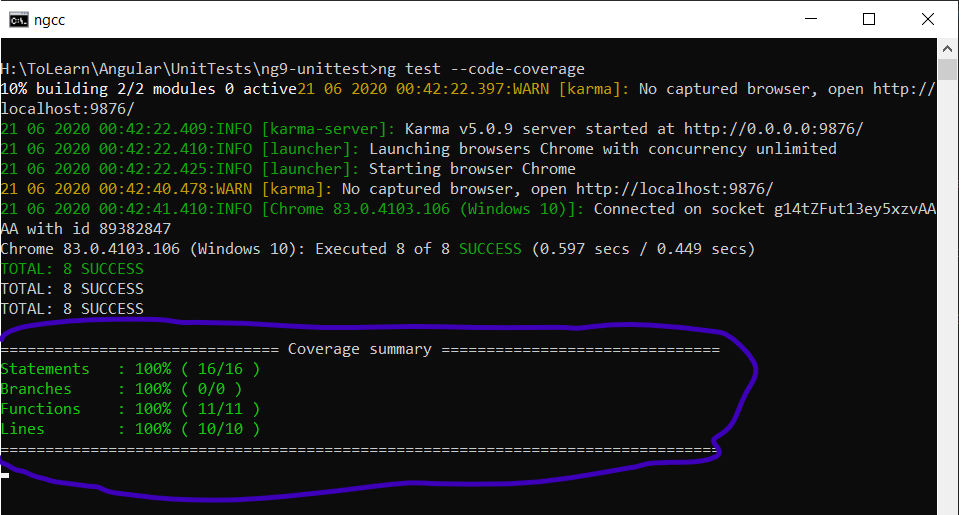
Yes there won’t be any difference in console view, but this time there will be a coverage folder created under project root path and if you open ./coverage/[projectname]/index.html it will show the detailed view of code coverage.
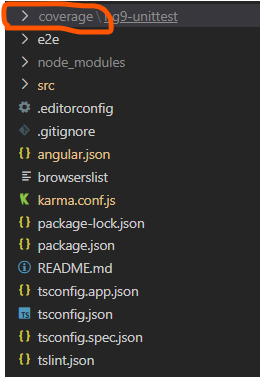
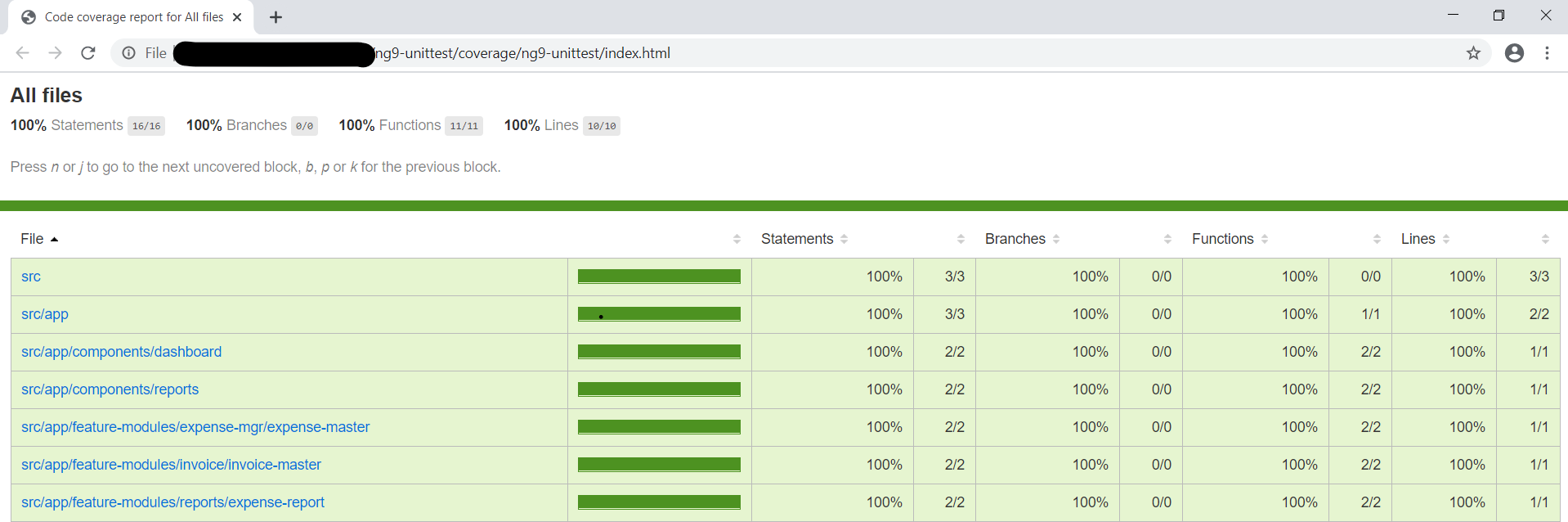
Configure specific folders for test execution
Assume for some reason, we don’t want to include/execute unit tests for our shared modules folder.
Let see how to do that, As we discussed before test.ts is the file where we configure our spec file context. Unfortunately, we can’t do it with a single statement.
We have to create multiple contexts for each folder where we can avoid /skip a shared folder.
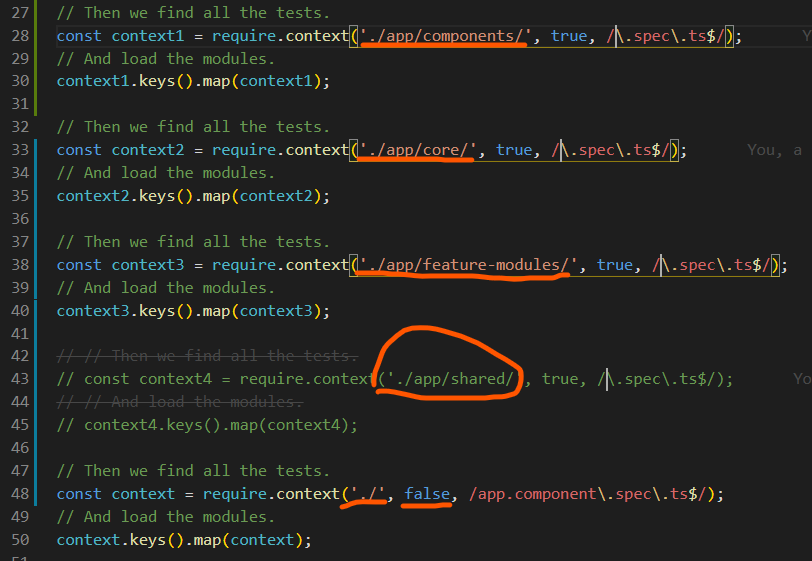
Here we are creating multiple context objects for each folder. Also, I have commented out the context for ./app/shared modules folder, hence the test cases won’t be executed for this module.
If you notice, for the last context object I’m passing the second parameters false (includeSubDirectories = false), this is important, if we pass this as true then all the folders inside the root will be executed.
NOTE: I have seen some people trying this with exclude option in tsconfig.spec.ts, as far as I know, that will just instruct the typescript compiler to exclude the compilation process for those files.